Well...I did it.
After months of sleepless nights and unnecessary perfectionism, I finally did it. I built my first website.
Originally I set out wanting to create a portfolio website for myself to showcase to friends, family, and most importantly, employers. I had never worked on a project like this before and thought it would also be a "fun challenge."
What I found out was that HTML sucks. I found myself annoyed at how often I had to copy and paste code, with almost no modularity (one of my favorite parts about programming). I knew there had to be a better way. So I shelved the project for another day.
Finally, in December of last year, I was wanting to customize my Mac. This led me to Übersicht which allowed Mac-users to create their own custom widgets. And these widgets were built using a little framework known as React (heard of it?). So I made my own (find it here) and started my journey of learning React.
And here we are, a few months later. So what did we learn?
The Great Gatsby
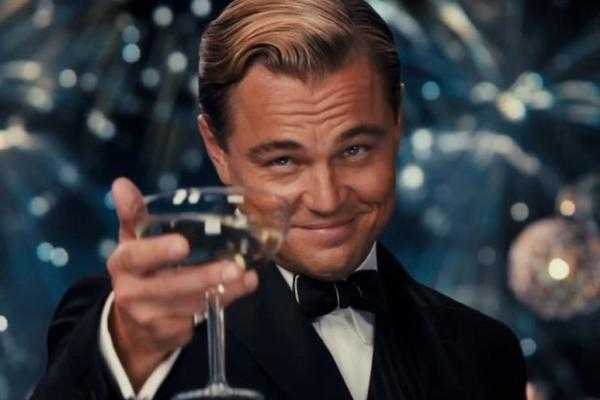
No not that Great Gatsby. This Great Gatsby:
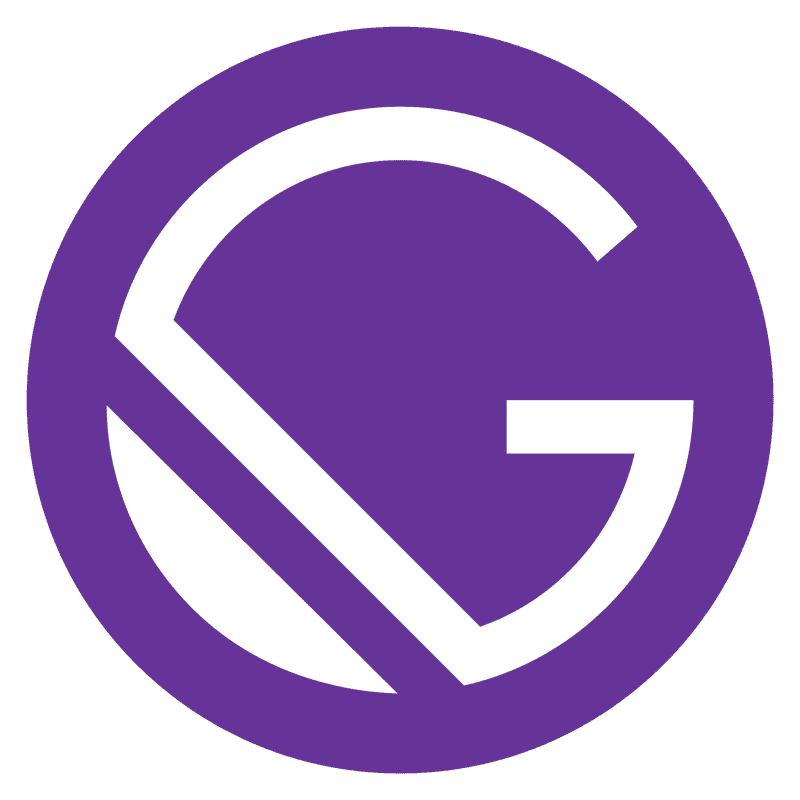
GatsbyJS was a huge help in building this website. It handled a lot of the backend stuff like routing and data management and was highly extensible with all of its community-created plugins. And best of all, it was totally free and open source!
I'm not working for them, I promise. I just really enjoyed their web framework. Of course, Gatsby is only as good as the person using it. And as I was learning how to use Gatsby, I was still learning...
React
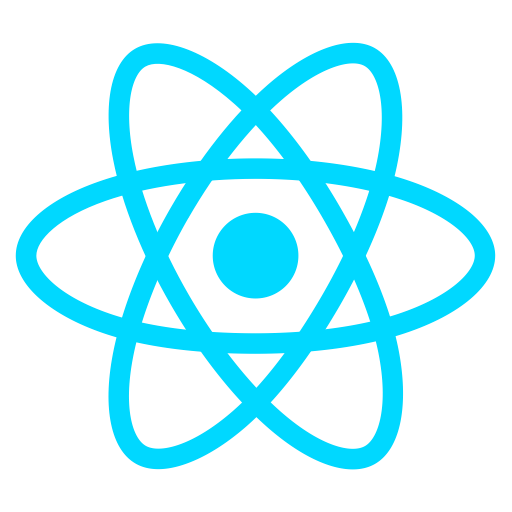
Even after creating that Übersicht widget, I still did not really understand React. I just knew that it could make my stupid HTML code more modular and therefore, more pleasing to debug and maintain. But learning React was one of the largest hurdles.
I worked on one page at a time, not moving onto the next until the current was in mostly-working condition. And there is a visible shift in code quality as I got better with my tools.
I struggled with understanding the Component life-cycle and what hooks even are. I was unfamiliar with composition and tried to work the framework into ways it didn't want to be used. At first, I hardly broke components down into smaller pieces. I chose React over basic HTML so that I could make my code more modular and easier to maintain. Do you see the irony here?
It took a lot of time to break this habit. One subtle change I made to help me do this was switching from Class Components to Functional Components. It might seem trivial, but I think thinking of components as functions helped me get over the idea that each component should be its own contained, ginormous beast of an object.
This change certainly helped me see how components worked. For example, I no longer had access to the mysterious componentDidMount
and comonentDidUpdate
methods. The questions of how they worked went with them. When does a component mount? How do I succinctly let these two share code? In its place came the useEffect
hook. And it taught me so much about why a component updates, when those changes take place, and how to keep the mounting/unmounting of a component legible by a human being.
Speaking of legibility...
Some Much Needed Structure
I like to think of myself as a "clean coder." I work on a problem, solve it, debug it, and then I try to always leave some documentation detailing how the code works, often more than needed.
However, with so much learning happening, I was constantly going back and fixing issues or updating code. The more I learned, the more the code changed. And the more the code changed, the less documentation there was. This was very frustrating when I would go back to old code in order to fix a bug or change something site-wide and I had no clue what was happening.
For example, I have these magic numbers that are the only ones that work and I have no clue why:
// The thresholds for the transformations
const mobility = {
up: {
rot: 35,
tran: 20,
},
down: {
rot: 25,
tran: 10,
},
left: {
rot: 15,
tran: 15,
},
right: {
rot: 15,
tran: 15,
},
}
On top of this, I was starting to get really messy with my organization. As I started to become more comfortable breaking things down into smaller components, I found that I had files all over the place and no real structure. It took me a good fifteen seconds to find a file sometimes.
Yes, my organization was severely hurting my efficiency. And it still is to an extent. I have yet to fully adopt a good commenting system. I am constantly changing things ever so slightly and I suppose at this point I'm too afraid of taking th time to document things. I have recently been doing a better job at it, so there may be hope just yet!
However, the file unstructure was getting out of hand. So I took some time to make this:
File Structure
==============
src
|-- pages/
| |-- about/
| | |-- index.js
| | |-- components/
| | | |-- component.js
. . . .
|-- data/
| |-- technologies.json
. .
|-- components/
| |-- ...
. .
|-- templates/
| |-- ...
. .
|-- sass/
| |-- config.scss
. .
|-- posts/
| |-- 2020-01-31--My-Post/
| | |-- index.md
. . .
|-- projects/
| |-- Avion/
| | |-- project.md
|-- assets/
| |-- icons/
| | |-- ...
| |-- images/
| | |-- ...
. . .
|-- utils/
| |-- tools/
| | |-- hash.js
| |-- setup/
| | |-- typography.js
| |-- animation/
| | |-- transitions.js
. . .
along with some detailed descriptions.
Once I created this and implemented it, navigating my codebase was much less of a daunting task. It's not perfect and it has certainly changed a bit since then, but it really allowed me to be more efficient.
One of my fears before redoing my file structure was that it wouldn't be "standard." I always take more time on stuff like this than I should because I don't want to put stuff in the src/
folder when everyone knows it should go in the lib/
folder. Silly things like that. But this time, I said, "You know what? My code, my rules." And it was very relieving to just do something without worrying about what make-believe people might see in my website's organization.
Design Woes
A smaller issue that I faced was in terms of design. I didn't want my site to feel like a cookie-cutter site. There's nothing wrong with those sites. In fact, they are cookie-cutter for a reason: they look great. But I didn't really care if my site looked "great," I just wanted to make it my own and maybe show my abilities. So I did just that.
Originally, I was going to do a pure video game theme, seeing as I want to get into the Games Industry. But I got some feedback and found that to be too limiting. After all, what if I really do end up loving development in React? So with only the homepage done, I decided to rethink my theme.
That's when my fiancée gave me a simple yet effective idea: technology. So with that, I got to work mapping things out in Figma.
With the Home page already a game controller, I planned for the Experience page to be a computer, the About page to be a phone, the Blog page to be film/television, and the Contact page to be radio/audio. But most importantly, I planned it to be fun and something I would enjoy working on.
After putting it in Figma and realizing it in code, I began to see the merits of the planning phase. Often times, I want to just rush to programming something but without that basis to go off of, I would be lost and months from finishing.
My Type of Code
The last major thing I learned through all of this was that JavaScript is kinda annoying.
What does this prop do again? Does this object have this key in it? Is this a number or a string?!
I used to think that dynamically typed languages were the best because I didn't have to write out stupid int
and float
anymore. And while I still enjoy not having to make distinctions like that a lot of the time, with certain situations it becomes a real pain— often felt through hours of debugging.
So I gave in. I decided I wanted to at least have the option to statically type my code.
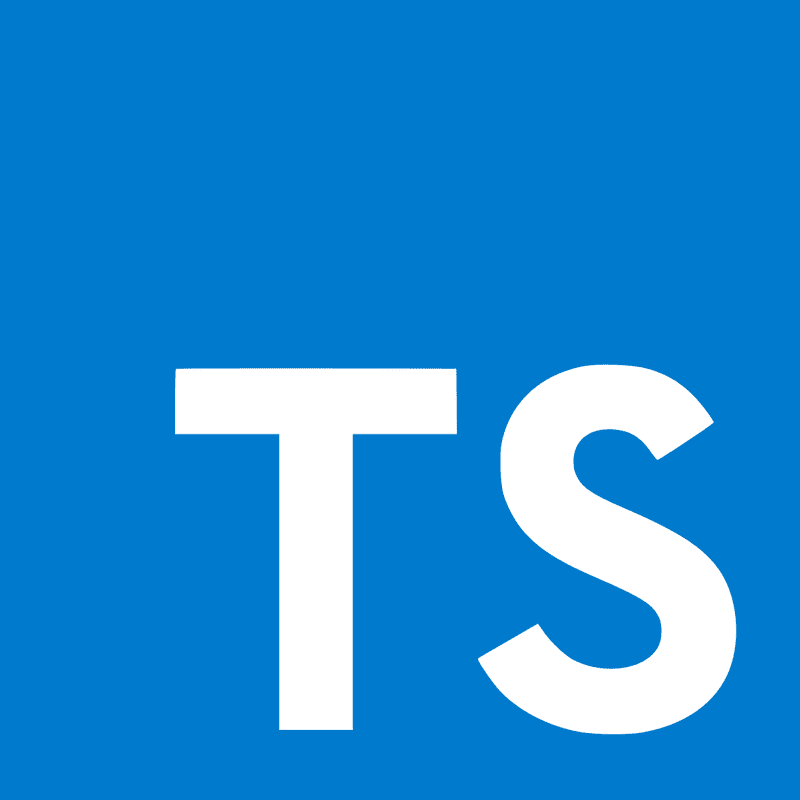
Enter TypeScript.
I had known about TypeScript for a while, but it often seemed like too much of a hassle to set up for whatever small projects I was working on. But I figured that this project was becoming large enough to warrant a switch.
Surprisingly, the switch was pretty easy. Gatsby handled the compilation and organization for me, all I had to do was configure my tsconfig.json
.
And I'm telling you, my eyes were opened. I could see clearly what everything did and what types a function expected. It even let me create my own interfaces to create my own types. I know I've only scratched the surface, but it is quite a step up from plain old JavaScript where everything is any
and nothing makes sense.
Moving Forward
I have definitely learned a lot from this project. I have also learned that there's still a lot left to learn for me. But I'm thankful I got to do it.
While I don't intend on always doing WebDev stuff, I think I found it to be more enjoyable than I anticipated. Maybe I'll make another site for fun one day. But for now, this one is plenty. And with lots of content to add and bugs to squish, I don't intend on replacing it so soon.
But I'll do that later. Time for a break.